How to Create a ZIP Archive with PHP
You can programmatically create Zip archives in your PHP web application or website using the functionality of one of the compression and archive extensions such as the Zip extension. In this tutorial, I will demonstrate how to create a Zip archive with PHP.
If you are operating a website or web application which provides file, software or image downloads and handles frequent downloads by users, then it might be a good idea to use some sort of zip archiving in order to save time (download time for the user) and bandwidth (both for the user and for you). Especially if you are offering multiple file downloads that have a high chance of being downloaded at the same time, offering them as a single Zip archive will be most efficient.
There are many cases where such a zipping feature may come handy. For example, you can think of how email service providers (e.g. Yahoo, Outlook) allow you to download multiple file attachments in an email as a Zip archive. Or, you can think of a dynamic template generation application that allows the user to download the template files after they are generated.
You can create Zip or other types of compressed archives with the help of a number of PHP extensions that you can find here. Since it is the most commonly used one, I will be using the Zip extension in this tutorial.
Now, let's start with the Zip archiving process.
STEP 1: Check if PHP's Zip Extension is Enabled
The first thing you need to do before starting is to check whether the Zip extension is enabled or not on your server. Depending on your server environment, your hosting plan and PHP version, you may or may not have the Zip extension installed or enabled. I have been working with PHP 5.6 for some time now and if I recall correctly, the Zip extension was built into it by default.
In any case, you can check your PHP details using the phpinfo() function and see if the Zip extension is enabled. If it is enabled, you should see something like the following on your PHP version details page:
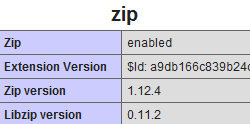
TIP: You can check your PHP version details by putting the following into an empty page and opening the page:
<?php phpinfo() ?>
TIP: You can also check whether a PHP extension is loaded or not using the extension_loaded() function, like the following:
<?php
if (extension_loaded('zip')) {
// Zip extension is enabled...
}
?>
STEP 2: Prepare the Files/Folder to be Zipped
You don't need to group or bring together the files and folders you are going to add to your zip archive but for the sake of simplicity, I will place all the files that will be zipped, in a separate folder which is named as example, like the following:
example/file1.txt
example/file2.txt
example/image.jpg
Our folder consists of two text files and one image file.
STEP 3: Add the Files to the Zip Archive
We can now create our Zip archive using a simple PHP script. The name of the PHP file will be zip.php and it will be located in the same directory as the example directory is.
We first define a zip object ($zip) using the ZipArchive class and specify a name and path to our zip archive file, like the following:
<?php
$zip = new ZipArchive;
$archive = 'archive.zip';
?>
Note that if you don't have the Zip extension enabled on your server, you will get an error. In the above example, we chose the path of the output Zip archive file (archive.zip) the same as the directory our script is located. You can specify its path as you wish depending on your needs.
Our next step is to check if the Zip archive can be successfully created. This check is not mandatory but it is better to have it just in case the zipping fails for any reason at the time of the script execution.
<?php
$zip = new ZipArchive;
$archive = 'archive.zip';
if ($zip->open($archive, ZipArchive::CREATE) !== TRUE) {
// Zip operation failed...
}
?>
What to do when the Zip operation fails is up to you; display an error notice, redirect the user to another page or do anything as it fits your design.
In case of success, we will start adding files to our Zip archive.
Zip a Single File with PHP
In the first example, we will compress a single file, in other words, we will add only one file to our Zip archive, using the addFile method of the ZipArchive class. The code we use for that is as follows:
<?php
$zip = new ZipArchive;
$archive = 'archive.zip';
if ($zip->open($archive, ZipArchive::CREATE) !== TRUE) {
// Zip operation failed...
} else {
$zip->addFile('example/file1.txt', 'file1.txt');
$zip->close();
}
?>
The first parameter of the addFile method is the path the file that will be added to the Zip archive. The second parameter is the (new) name of that file in the archive.
Once the file is added, we close the $zip object and finalize its creation.
If you followed all the steps carefully so far, once you run this script on your server, you should see a new zip archive, archive.zip, in the same directory where the example folder and zip.php file are located.
Zip Multiple Files with PHP
Following the same concept, you can add multiple files into your Zip archive using the code below. This time, I used new names for the files that are added to the archive.
<?php
$zip = new ZipArchive;
$archive = 'archive.zip';
if ($zip->open($archive, ZipArchive::CREATE) !== TRUE) {
// Zip operation failed...
} else {
$zip->addFile('example/file1.txt', 'myfile-1.txt');
$zip->addFile('example/file2.txt', 'myfile-2.txt');
$zip->addFile('example/image.jpg', 'myimage.jpg');
$zip->close();
}
?>
You can check the created archive to see how the new names are applied.
Zip All Files in a Folder with PHP
If you have a folder with unknown number of files in it and you want to add all of them to the Zip archive, you can get all the files in the folder and add them using a loop.
<?php
$zip = new ZipArchive;
$archive = 'archive.zip';
if ($zip->open($archive, ZipArchive::CREATE) !== TRUE) {
// Zip operation failed...
} else {
$files = glob('example/*');
foreach ($files as $file) {
$zip->addFile($file, str_replace('example/', '', $file));
}
$zip->close();
}
?>
We got all the files using the glob() function, which returns the file paths (what addFile() uses as the first parameter), and then we used str_replace() to remove the folder name from the file paths.
More PHP Tips
How to Create a Cookie with PHP How to Delete a Cookie with PHP How to Download a File with PHP How to Include PHP Variables in JavaScript How to Get All Files and Folders in a Folder with PHP How to Control CSS Variables with PHP How to Get the Subfolders in a Folder with PHP Facebook Share Button Code with PHP How to Get the Number of Files in a Folder with PHP How to Get All Files in a Folder with PHP
PHP Tips