PHP Image Watermark: How to Add a Watermark to Images in PHP
It is possible to programmatically add a watermark (by using a watermark image) to your images with PHP. In this tutorial, I will demonstrate how to do that by giving an example.
A watermark is like a new transparent layer on your image, which isn't a part of the original image but that provides additional information about the image or the creator/owner of it. You may need to add a watermark sign to your images on your websites or applications for a number of reasons such as preventing unauthorized usage or marketing of your website by placing your URL or logo on your images so that anyone that sees your images online (e.g. on search engines, on Pinterest) will know their source.
You can use image editing software such as Photoshop or GIMP to place watermarks on your images manually. Often times, the ability to watermark an image via an image editing software will be enough if you are working on a small number of images or you don't need to do it automatically. However, if you need this ability on your website or web application either to put a watermark on a large number of images in multiple directories programmatically or to automatically use it on images that your users upload to your website, you can use PHP's image creation and manipulation features to accomplish this.
In order to put a watermark on another image, you need to use a transparent watermark image. Note that, JPEG (or JPG) images cannot be used as watermark since they have no transparency (alpha channel). You will need to use either PNG or GIF type of images as the watermark. My recommendation will be to use PNG, which I will be using in the example below.
PHP GD Extension
PHP has a number of extensions (libraries) that provide image creation and manipulation capabilities such as GD (Graphics Draw), Gmagick and ImageMagick. In this example, I will make use of the GD extension. You can work with JPEG, PNG and GIF image types with GD.
Remember that PHP extensions need to be installed and enabled in order to function. Before continuing with the rest of this tutorial, please make sure that the GD extension is installed and enabled on your server.
Adding a Watermark to an Image with PHP
Now, let's continue with placing a watermark on an image with the help of a PHP script. Naturally, we will need an image to be watermarked and a watermark image. I will use the following JPG image as the source image:
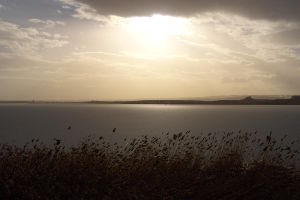
And I will use the following transparent PNG image as the watermark image:
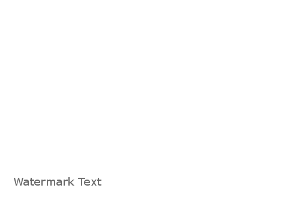
Our desired output is that the watermark text will be merged on the source image and the result will be displayed on our page as a single JPEG image file. To make things simpler, I created the watermark image with the same dimensions (width & height) as the source image. You can also use a watermark image with different dimensions and I will cover that in another tutorial.
STEP 1: Define the source image and the watermark image.
Create a new text file and name it as watermark.php, or anything you want, and put the following code into this file:
<?php
$image = imagecreatefromjpeg('image.jpg');
$watermark = imagecreatefrompng('watermark.png');
?>
What we did is that we created a new image with $image variable and assigned the source image (image.jpg) to it using the imagecreatefromjpeg() function of GD extension and we created another image with $watermark variable and assigned the watermark image (watermark.png) to it using the imagecreatefrompng() function.
In this example, watermark.php, image.jpg and watermark.png files are in the same directory. If your images are located in a different directory, simply insert their paths.
STEP 2: Merge the watermark with the source image.
With the two new images created, our next step is to merge them so that the watermark text will be added to the original image. The merge process is executed with the help of the imagecopy() function.
Our code should now look like this:
<?php
$image = imagecreatefromjpeg('image.jpg');
$watermark = imagecreatefrompng('watermark.png');
imagecopy($image, $watermark, 0, 0, 0, 0, imagesx($watermark), imagesy($watermark));
?>
The watermark is now merged onto the source image.
I will briefly mention what the parameters of the imagecopy() function are. The first parameter ($image) is the original image that the watermark will be placed on. The second parameter ($watermark) is the watermark image. The next four parameters (0) are x- and y- coordinates of the original image and the watermark respectively. I am not going into the details about the coordinates here because our images have the same dimensions, and also I will further discuss about the coordinates in another tutorial where I will add a watermark with different dimensions. The last two parameters (imagesx($watermark) and imagesy($watermark)) are the width and height of the watermark image.
STEP 3: Display the watermarked image.
Now that our watermarked image is ready, we can display it. Update the code in the watermark.php file as follows:
<?php
$image = imagecreatefromjpeg('image.jpg');
$watermark = imagecreatefrompng('watermark.png');
imagecopy($image, $watermark, 0, 0, 0, 0, imagesx($watermark), imagesy($watermark));
header('Content-Type: image/jpeg');
imagejpeg($image);
imagedestroy($image);
?>
In the above code, header() sends the content type header information to the browser, in our case it is a JPEG image file. imagejpeg() outputs the resulting image, in this case to the browser. imagedestroy(), which is optional, removes the image from memory by destroying it.
When you open the watermark.php file in your browser now, you will see the watermarked image like the following:
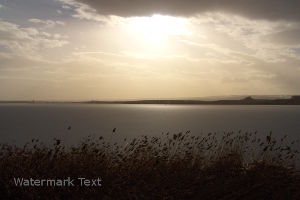
Displaying the resulting image in the browser is the first and simplest thing that we can do after adding the watermark to it. I will cover other points such as saving the resulting image to the server, adding a watermark to multiple images in multiple directories or applying the watermark during user upload in another post.
More PHP Tips
Simple PHP-MySQL Database Connection Class Using mysqli How to Get All Files in a Folder with PHP How to Get the Number of Files in a Folder with PHP How to Get the Subfolders in a Folder with PHP How to Control CSS Variables with PHP How to Get All Files and Folders in a Folder with PHP How to Include PHP Variables in JavaScript How to Download a File with PHP How to Create a ZIP Archive with PHP How to Create a Cookie with PHP
PHP Tips